
The reason why there’s no unzip() function in Python is because the opposite of zip() is… well, zip(). There’s a question that comes up frequently in forums for new Pythonistas: “If there’s a zip() function, then why is there no unzip() function that does the opposite?” If you forget this detail, the final result of your program may not be quite what you want or expect. However, you’ll need to consider that, unlike dictionaries in Python 3.6, sets don’t keep their elements in order. You can also use Python’s zip() function to iterate through sets in parallel. Notice that, in the above example, the left-to-right evaluation order is guaranteed.
DIFFERENCE BETWEEN ZIP AND IZIP PYTHON HOW TO
Note: If you want to dive deeper into dictionary iteration, check out How to Iterate Through a Dictionary in Python. It produces the same effect as zip() in Python 3:
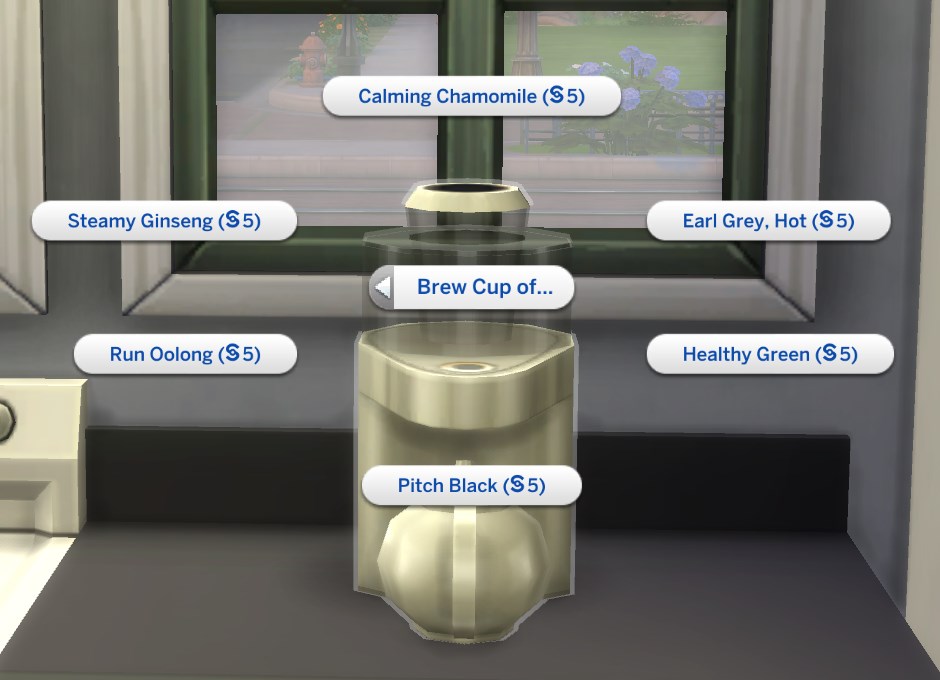
This function creates an iterator that aggregates elements from each of the iterables. In these situations, consider using itertools.izip(*iterables) instead. If you regularly use Python 2, then note that using zip() with long input iterables can unintentionally consume a lot of memory. This will run through the iterator and return a list of tuples. In Python 3, you can also emulate the Python 2 behavior of zip() by wrapping the returned iterator in a call to list(). The first iteration is truncated at C, and the second one results in a StopIteration exception. Here, your call to zip() returns an iterator.

# Python 3 > zipped = zip ( range ( 3 ), 'ABCD' ) > zipped # Hold an iterator > type ( zipped ) > list ( zipped ) > zipped = zip () # Create an empty iterator > zipped > next ( zipped ) Traceback (most recent call last):įile "", line 1, in next ( zipped ) StopIteration
